일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 설치
- 서버
- packet-tracer
- 설정
- comand
- 이클립스
- configration
- 언어
- board
- 게시판
- tomcat
- CLI
- server
- 넷플릭스속도테스트
- JSP
- 단축키
- 패킷트레이서
- war
- vmware
- java
- cisco
- 시스코
- 한글
- 무농약
- eclipse
- centos
- centos7
- interface
- 홈가든
- servlet
- Today
- Total
넓고얕은지식사전
JSP & Servlet 게시판 구현하기(게시글 목록 조회) 본문
지난번에 게시글 쓰기 기능을 구현 하였다.
https://nullgoyatten.tistory.com/22
JSP & Servlet 게시판 구현하기(게시글 쓰기)
지난번에 암호변경 기능을 구현 하였다. https://nullgoyatten.tistory.com/21 JSP & Servlet 게시판 구현하기(암호 변경) 지난번에 로그아웃 기능을 구현 하였다. https://nullgoyatten.tistory.com/20 JSP & Servlet 게시판
nullgoyatten.tistory.com
이번에는 게시글 목록 조회 기능을 구현하도록 하자.
1. ArticleDao 에 selectCount 메서드 추가 (전체 게시글 개수를 구하기 위함)
경로 : article.dao> ArticleDao.java
public int selectCount(Connection conn) throws SQLException {
Statement stmt = null;
ResultSet rs = null;
try {
stmt = conn.createStatement();
rs = stmt.executeQuery("select count(*) from article");
if (rs.next()) {
return rs.getInt(1);
}
return 0;
} finally {
JdbcUtil.close(rs);
JdbcUtil.close(stmt);
}
}
2. ArticleDao 에 select메서드 추가(지정한 범위의 게시글을 읽어오기 위함)
위의
경로 : article.dao> ArticleDao.java
public List<Article> select(Connection conn, int startRow, int size) throws SQLException {
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
pstmt = conn.prepareStatement("select * from article " +
"order by article_no desc limit ?, ?");
pstmt.setInt(1, startRow);
pstmt.setInt(2, size);
rs = pstmt.executeQuery();
List<Article> result = new ArrayList<>();
while (rs.next()) {
result.add(convertArticle(rs));
}
return result;
} finally {
JdbcUtil.close(rs);
JdbcUtil.close(pstmt);
}
}
private Article convertArticle(ResultSet rs) throws SQLException {
return new Article(rs.getInt("article_no"),
new Writer(
rs.getString("writer_id"),
rs.getString("writer_name")),
rs.getString("title"),
toDate(rs.getTimestamp("regdate")),
toDate(rs.getTimestamp("moddate")),
rs.getInt("read_cnt"));
}
private Date toDate(Timestamp timestamp) {
return new Date(timestamp.getTime());
}
}
3. 위 두 메서드를 추가한 전체 코드는 아래와 같다.
package article.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.sql.Timestamp;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import article.model.Article;
import article.model.Writer;
import jdbc.JdbcUtil;
public class ArticleDao {
public Article insert(Connection conn, Article article) throws SQLException {
PreparedStatement pstmt = null;
Statement stmt = null;
ResultSet rs = null;
try {
pstmt = conn.prepareStatement("insert into article "
+ "(writer_id, writer_name, title, regdate, moddate, read_cnt) "
+ "values (?,?,?,?,?,0)");
pstmt.setString(1, article.getWriter().getId());
pstmt.setString(2, article.getWriter().getName());
pstmt.setString(3, article.getTitle());
pstmt.setTimestamp(4, toTimestamp(article.getRegDate()));
pstmt.setTimestamp(5, toTimestamp(article.getModifiedDate()));
int insertedCount = pstmt.executeUpdate();
if (insertedCount > 0) {
stmt = conn.createStatement();
rs = stmt.executeQuery("select last_insert_id() from article");
if (rs.next()) {
Integer newNo = rs.getInt(1);
return new Article(newNo,
article.getWriter(),
article.getTitle(),
article.getRegDate(),
article.getModifiedDate(),
0);
}
}
return null;
} finally {
JdbcUtil.close(rs);
JdbcUtil.close(stmt);
JdbcUtil.close(pstmt);
}
}
private Timestamp toTimestamp(Date date) {
return new Timestamp(date.getTime());
}
public int selectCount(Connection conn) throws SQLException {
Statement stmt = null;
ResultSet rs = null;
try {
stmt = conn.createStatement();
rs = stmt.executeQuery("select count(*) from article");
if (rs.next()) {
return rs.getInt(1);
}
return 0;
} finally {
JdbcUtil.close(rs);
JdbcUtil.close(stmt);
}
}
public List<Article> select(Connection conn, int startRow, int size) throws SQLException {
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
pstmt = conn.prepareStatement("select * from article " +
"order by article_no desc limit ?, ?");
pstmt.setInt(1, startRow);
pstmt.setInt(2, size);
rs = pstmt.executeQuery();
List<Article> result = new ArrayList<>();
while (rs.next()) {
result.add(convertArticle(rs));
}
return result;
} finally {
JdbcUtil.close(rs);
JdbcUtil.close(pstmt);
}
}
private Article convertArticle(ResultSet rs) throws SQLException {
return new Article(rs.getInt("article_no"),
new Writer(
rs.getString("writer_id"),
rs.getString("writer_name")),
rs.getString("title"),
toDate(rs.getTimestamp("regdate")),
toDate(rs.getTimestamp("moddate")),
rs.getInt("read_cnt"));
}
private Date toDate(Timestamp timestamp) {
return new Date(timestamp.getTime());
}
}
4. ArticlePage 작성 (게시글 목록을 제공하는 서비스 클래스를 구현하기 위해 데이터와 페이징 정보를 담을 곳이 필요하다.)
경로 : article.service > ArticlePage.java
package article.service;
import java.util.List;
import article.model.Article;
public class ArticlePage {
private int total;
private int currentPage;
private List<Article> content;
private int totalPages;
private int startPage;
private int endPage;
public ArticlePage(int total, int currentPage, int size, List<Article> content) {
this.total = total;
this.currentPage = currentPage;
this.content = content;
if (total == 0) {
totalPages = 0;
startPage = 0;
endPage = 0;
} else {
totalPages = total / size;
if (total % size > 0) {
totalPages++;
}
int modVal = currentPage % 5;
startPage = currentPage / 5 * 5 + 1;
if (modVal == 0) startPage -= 5;
endPage = startPage + 4;
if (endPage > totalPages) endPage = totalPages;
}
}
public int getTotal() {
return total;
}
public boolean hasNoArticles() {
return total == 0;
}
public boolean hasArticles() {
return total > 0;
}
public int getCurrentPage() {
return currentPage;
}
public int getTotalPages() {
return totalPages;
}
public List<Article> getContent() {
return content;
}
public int getStartPage() {
return startPage;
}
public int getEndPage() {
return endPage;
}
}
5. ListArticleService 작성 (게시글 목록을 제공하는 서비스 클래스를 구현.)
경로 : article.service > ListArticleService.java
package article.service;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
import article.dao.ArticleDao;
import article.model.Article;
import jdbc.connection.ConnectionProvider;
public class ListArticleService {
private ArticleDao articleDao = new ArticleDao();
private int size = 10;
public ArticlePage getArticlePage(int pageNum) {
try (Connection conn = ConnectionProvider.getConnection()) {
int total = articleDao.selectCount(conn);
List<Article> content = articleDao.select(
conn, (pageNum - 1) * size, size);
return new ArticlePage(total, pageNum, size, content);
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
}
6. ListArticleHandler 작성
경로 : article.command > ListArticleHandler.java
package article.command;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import article.service.ArticlePage;
import article.service.ListArticleService;
import mvc.command.CommandHandler;
public class ListArticleHandler implements CommandHandler {
private ListArticleService listService = new ListArticleService();
@Override
public String process(HttpServletRequest req, HttpServletResponse res)
throws Exception {
String pageNoVal = req.getParameter("pageNo");
int pageNo = 1;
if (pageNoVal != null) {
pageNo = Integer.parseInt(pageNoVal);
}
ArticlePage articlePage = listService.getArticlePage(pageNo);
req.setAttribute("articlePage", articlePage);
return "/WEB-INF/view/listArticle.jsp";
}
}
7. commandHandlerURI.properties 수정. ListArticleHandler가 /article/list.do 요청을 처리하도록 매핑 설정에 추가한다.
/join.do=member.command.JoinHandler
/login.do=auth.command.LoginHandler
/logout.do=auth.command.LogoutHandler
/changePwd.do=member.command.ChangePasswordHandler
/article/write.do=article.command.WriteArticleHandler
/article/list.do=article.command.ListArticleHandler
8. listArticle.jsp 작성
경로 : WebContent > WEB-INF > view > listArticle.jsp
<%@ page language="java" contentType="text/html; charset=EUC-KR"
pageEncoding="EUC-KR"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=EUC-KR">
<title>게시글 목록</title>
</head>
<body>
<table border="1">
<tr>
<td colspan="4"><a href="write.do">[게시글쓰기]</a></td>
</tr>
<tr>
<td>번호</td>
<td>제목</td>
<td>작성자</td>
<td>조회수</td>
</tr>
<c:if test="${articlePage.hasNoArticles()}">
<tr>
<td colspan="4">게시글이 없습니다.</td>
</tr>
</c:if>
<c:forEach var="article" items="${articlePage.content}">
<tr>
<td>${article.number}</td>
<td>
<a href="read.do?no=${article.number}&pageNo=${articlePage.currentPage}">
<c:out value="${article.title}"/>
</a>
</td>
<td>${article.writer.name}</td>
<td>${article.readCount}</td>
</tr>
</c:forEach>
<c:if test="${articlePage.hasArticles()}">
<tr>
<td colspan="4">
<c:if test="${articlePage.startPage > 5}">
<a href="list.do?pageNo=${articlePage.startPage - 5}">[이전]</a>
</c:if>
<c:forEach var="pNo"
begin="${articlePage.startPage}"
end="${articlePage.endPage}">
<a href="list.do?pageNo=${pNo}">[${pNo}]</a>
</c:forEach>
<c:if test="${articlePage.endPage < articlePage.totalPages}">
<a href="list.do?pageNo=${articlePage.startPage + 5}">[다음]</a>
</c:if>
</td>
</tr>
</c:if>
</table>
</body>
</html>
9. web.xml 수정
web.xml(경로 : WebContent > WEB-INF)에 LoginCheckFilter 설정(list.do)을 추가해줘야 한다.
<!-- LoginCheckFilter관련 설정 -->
<filter>
<filter-name>LoginCheckFilter</filter-name>
<filter-class>filter.LoginCheckFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>LoginCheckFilter</filter-name>
<url-pattern>/changePwd.do</url-pattern>
<url-pattern>/article/list.do</url-pattern>
<url-pattern>/article/write.do</url-pattern>
</filter-mapping>
<!-- LoginCheckFilter관련 설정 -->
10. 테스트(http://localhost:8080/board/article/list.do)
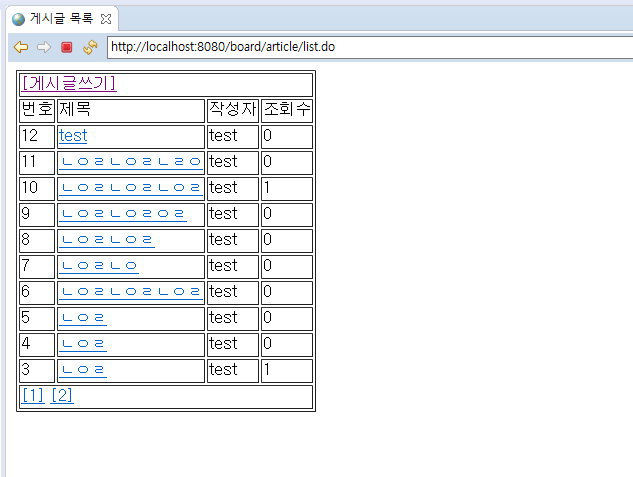
다음글 : 게시글 내용 조회 구현
https://nullgoyatten.tistory.com/26
JSP & Servlet 게시판 구현하기(게시글 내용 조회)
지난번에 게시글 목록 조회 기능을 구현 하였다. 이번엔 작성된 내용 조회를 구현해 보도록 하자 1. ArticleDao 에 게시글 조회 관련 기능 추가 추가 (특정번호에 해당하는 게시글 데이터 읽기, 조회
nullgoyatten.tistory.com
'IT > JSP & Servlet' 카테고리의 다른 글
JSP & Servlet 게시판 구현하기(게시글 내용 수정) (0) | 2023.02.06 |
---|---|
JSP & Servlet 게시판 구현하기(게시글 내용 조회) (0) | 2023.02.06 |
JSP & Servlet 게시판 구현하기(게시글 쓰기) (0) | 2023.01.14 |
JSP & Servlet 게시판 구현하기(암호 변경) (0) | 2023.01.14 |
JSP & Servlet 게시판 구현하기(로그아웃) (0) | 2023.01.13 |